Extended sessions
An extended session is a period beyond the official trading hours of the exchange when trading still occurs. The library allows you to display extended sessions for symbols that support them. Note that extended sessions are only visible on intraday resolutions.
The extended session includes regular, pre-market, and post-market subsessions. Either a pre-market or post-market subsession can be missed. When the chart displays an extended session, the subsessions are represented as colored areas.
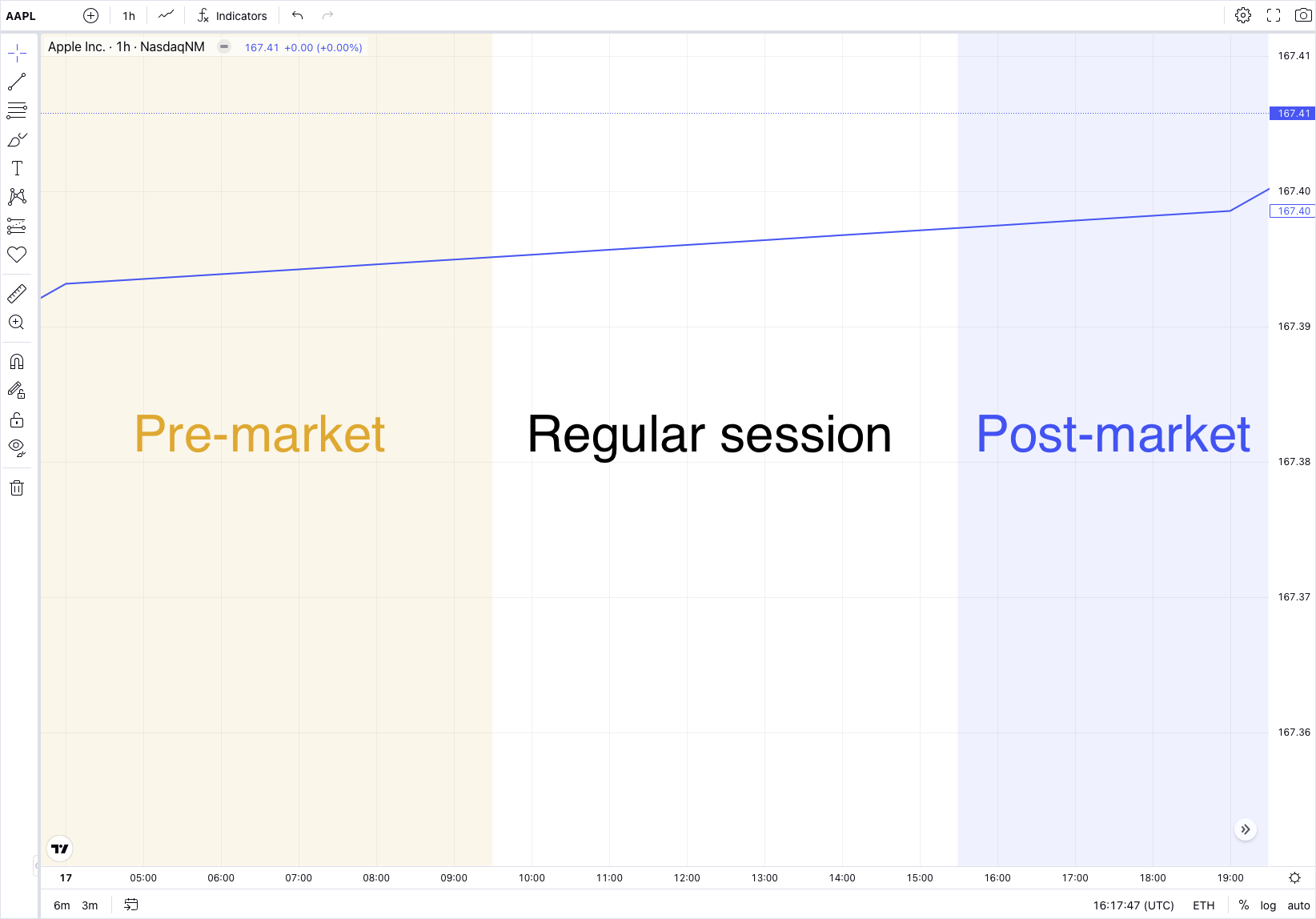
Enable extended sessions
To enable extended sessions, do the following:
- Add the
pre_post_market_sessions
featureset to theenabled_features
array. - Specify the
subsession_id
andsubsessions
properties inLibrarySymbolInfo
for symbols that support extended sessions. Refer to the Configure datafeed section for more information.
Specify the default session type
The chart can display regular or extended sessions. To specify the default session type, provide the mainSeriesProperties.sessionId
property within overrides
.
var widget = window.tvWidget = new TradingView.widget({
// ...
overrides: {
"mainSeriesProperties.sessionId": "extended",
}
});
Specify subsession colors
Subsessions are represented on the chart as colored areas. You can specify default colors for pre-market and post-market subsessions using the overrides
property in the Widget Constructor.
var widget = window.tvWidget = new TradingView.widget({
// ...
overrides: {
"backgrounds.preMarket.color": "rgba(200,0,0,0.08)",
"backgrounds.postMarket.color": "rgba(0,0,200,0.08)",
}
});
To change the colors on the fly, use the applyOverrides
method.
widget.applyOverrides({"backgrounds.outOfSession.color": "rgba(0,0,0,0.2)"});
widget.applyOverrides({"backgrounds.preMarket.color": "rgba(200,0,0,0.08)"});
widget.applyOverrides({"backgrounds.postMarket.color": "rgba(0,0,200,0.08)"});
Users can adjust the subsession colors in the Chart settings dialog in the UI.
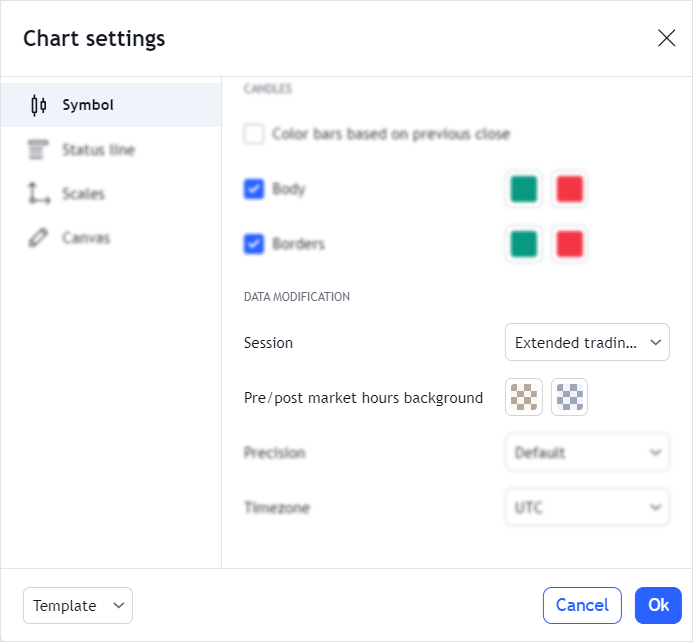
Change the session type
You can switch between the sessions in the UI or using the API.
In UI
Users can change the session type in the following ways:
-
Use the drop-down menu on the bottom toolbar.
-
Use the drop-down menu in the Chart settings dialog.
Using API
You can use the applyOverrides
method to change the session type on the fly.
widget.applyOverrides({ "mainSeriesProperties.sessionId": "extended" });
Configure the datafeed
To display extended sessions on the chart, you should provide additional data in LibrarySymbolInfo
.
LibrarySymbolInfo
is an object that contains information about a certain symbol. You should return this object to the library when it calls the resolveSymbol
method. Refer to Symbology for more information on how to implement LibrarySymbolInfo
.
Consider the LibrarySymbolInfo
object for a symbol that supports a regular session only.
{
"name": "AAPL",
"ticker": "AAPL",
"exchange": "NasdaqNM",
"timezone": "Etc/UTC",
"description": "Apple Inc.",
"type": "stock",
"pricescale": 100,
"minmov": 1,
"has_intraday": true,
"supported_resolutions": ["60"],
"has_daily": false,
"intraday_multipliers": ["1"],
"format": "price",
"session": "0930-1600"
}
If the symbol supports an extended session, you should additionally specify the subsession_id
and subsessions
properties.
The code sample below specifies an extended session that starts at 04:00 and ends at 20:00. It includes a pre-market subsession from 04:00 to 09:30, a regular subsession from 09:30 to 16:00, and a post-market subsession from 16:00 to 20:00.
{
"name": "AAPL",
"ticker": "AAPL",
"exchange": "NasdaqNM",
"timezone": "Etc/UTC",
"description": "Apple Inc.",
"type": "stock",
"pricescale": 100,
"minmov": 1,
"has_intraday": true,
"supported_resolutions": ["60"],
"has_daily": false,
"intraday_multipliers": ["1"],
"format": "price",
"session": "0930-1600",
"subsession_id": "regular",
"subsessions": [
{
"description": "Regular Trading Hours",
"id": "regular",
"session": "0930-1600"
},
{
"description": "Extended Trading Hours",
"id": "extended",
"session": "0400-2000"
},
{
"description": "Pre-market",
"id": "premarket",
"session": "0400-0930"
},
{
"description": "Post-market",
"id": "postmarket",
"session": "1600-2000"
}
]
}
Note that the session
and subsession_id
values depend on the current session type selected on the chart.
If the session type is changed either in the UI or using the API, you should update the LibrarySymbolInfo
object.
Handle session switch
When the session type is changed, the library needs to request all data from scratch.
To do this, the library calls resolveSymbol
once again with an additional SymbolResolveExtension
parameter. In SymbolResolveExtension
, the session
property indicates what session type should be displayed on the chart.
In response, you should return the updated LibrarySymbolInfo
object with the following properties changed:
subsession_id
: the property's value should match theSymbolResolveExtension.session
value. The value is either"regular"
or"extended"
.session
: the property's value should match the correspondingLibrarySubsessionInfo.session
value specified insubsessions
. For example, if the chart displays"extended"
session, and for this session typeLibrarySubsessionInfo.session
is"0400-2000"
, theLibrarySymbolInfo.session
value should also be"0400-2000"
.
You can use the following expression to make sure that session
is correct. The expression should always be true
:
symbolInfo.session === symbolInfo.subsessions.find(x => x.id === subsession_id).session
Example
Consider the example. The current symbol on the chart is AAPL
and the session type is regular. You should return the following LibrarySymbolInfo
object to the library:
{
"name": "AAPL",
"ticker": "AAPL",
"exchange": "NasdaqNM",
"timezone": "Etc/UTC",
"description": "Apple Inc.",
"type": "stock",
"pricescale": 100,
"minmov": 1,
"has_intraday": true,
"supported_resolutions": ["60"],
"has_daily": false,
"intraday_multipliers": ["1"],
"format": "price",
"session": "0930-1600",
"subsession_id": "regular",
"subsessions": [
{
"description": "Regular Trading Hours",
"id": "regular",
"session": "0930-1600"
},
{
"description": "Extended Trading Hours",
"id": "extended",
"session": "0400-2000"
},
{
"description": "Pre-market",
"id": "premarket",
"session": "0400-0930"
},
{
"description": "Post-market",
"id": "postmarket",
"session": "1600-2000"
}
]
}
Then, the chart is switched to the extended session. The library calls resolveSymbol
once again.
In response, you should update the subsession_id
and session
properties, so they match the corresponding values defined for the extended session in subsessions
:
{
"name": "AAPL",
"ticker": "AAPL",
"exchange": "NasdaqNM",
"timezone": "Etc/UTC",
"description": "Apple Inc.",
"type": "stock",
"pricescale": 100,
"minmov": 1,
"has_intraday": true,
"supported_resolutions": ["60"],
"has_daily": false,
"intraday_multipliers": ["1"],
"format": "price",
"session": "0400-2000", // The value is changed
"subsession_id": "extended", // The value is changed
"subsessions": [
{
"description": "Regular Trading Hours",
"id": "regular",
"session": "0930-1600"
},
{
"description": "Extended Trading Hours",
"id": "extended",
"session": "0400-2000"
},
{
"description": "Pre-market",
"id": "premarket",
"session": "0400-0930"
},
{
"description": "Post-market",
"id": "postmarket",
"session": "1600-2000"
}
]
}
Corrections for extended sessions
Corrections are changes in the default trading session for specific days.
For regular sessions, you should only specify the corrections
property in LibrarySymbolInfo
.
However, for extended sessions, you should additionally provide session-correction
for each subsession in subsessions
.
For example, you need to display the following sessions:
- Regular. The default regular session starts at 09:30 and ends at 17:00. On 2019‑07‑03, 2019‑11‑29, and 2019‑12‑24, the session starts at 09:30 and ends at 13:00.
- Extended. The default extended session starts at 04:00 and ends at 20:00. On 2019‑07‑03, 2019‑11‑29, and 2019‑12‑24, the session starts at 04:00 and ends at 21:00.
- Pre-market. This session always starts at 04:00 and ends at 09:30.
- Post-market. The default post-market session starts at 17:00 and ends at 20:00. However, on 2019‑07‑03, 2019‑11‑29, and 2019‑12-24, the session time shifts because of the changes in the regular and extended sessions. On these days, the post-market session starts at 13:00 and ends at 21:00.
{
// ...
"subsessions": [
{
"description": "Regular Trading Hours",
"id": "regular",
"session": "0930-1700",
"session-correction": "0930-1300:20190703,20191129,20191224",
},
{
"description": "Extended Trading Hours",
"id": "extended",
"session": "0400-2000",
"session-correction": "0400-2100:20190703,20191129,20191224",
},
{
"description": "Pre-market",
"id": "premarket",
"session": "0400-0930",
},
{
"description": "Post-market",
"id": "postmarket",
"session": "1700-2000",
"session-correction": "1300-2100:20190703,20191129,20191224",
}
],
}
Time changes in one session may cause changes in the related sessions. Make sure you specify corrections for all sessions that require them to avoid visual bugs.
As mentioned earlier, you should update the session
and session_id
properties in LibrarySymbolInfo
when the session type is changed.
If you specify corrections, you should also update the corrections
property.
This property should contain one of the session-correction
values, depending on the currently selected session type in the UI.
For example, the current type is "regular"
, therefore, corrections
is equal to the session-correction
value specified for the regular session.
{
// ...
"session": "0930-1700",
"subsession_id": "regular",
"corrections": "0930-1300:20190703,20191129,20191224",
"subsessions": [
{
"description": "Regular Trading Hours",
"id": "regular",
"session": "0930-1700",
"session-correction": "0930-1300:20190703,20191129,20191224",
},
{
"description": "Extended Trading Hours",
"id": "extended",
"session": "0400-2000",
"session-correction": "0400-2100:20190703,20191129,20191224",
},
// ...
],
}
If the type is changed to "extended"
, you should update corrections
to match the session-correction
value specified for the extended session.
{
// ...
"session": "0400-2000",
"subsession_id": "extended",
"corrections": "0400-2100:20190703,20191129,20191224",
"subsessions": [
{
"description": "Regular Trading Hours",
"id": "regular",
"session": "0930-1700",
"session-correction": "0930-1300:20190703,20191129,20191224",
},
{
"description": "Extended Trading Hours",
"id": "extended",
"session": "0400-2000",
"session-correction": "0400-2100:20190703,20191129,20191224",
},
// ...
],
}
Enable the price line
This feature is only available in Trading Platform as it requires quote data.
The library allows you to display pre-/post-market price lines. These lines appear only during the pre-/post-market sessions when the chart shows only the regular session. However, if the chart is set to display the extended session, the lines will not be visible, as the data is already displayed on the chart.

To enable the lines, you should do the following:
- Enable the
pre_post_market_price_line
featureset. - Provide the
rtc
property in theDatafeedQuoteValues
object.
You can also display information on the pre-/post-market price in Details.
To do this, you should additionally provide the rch
, rchp
, and rtc_time
properties in the DatafeedQuoteValues
object.
